Introduction
Do you want to activate an output of your industrial Raspberry Pi PLC and to know how long it will take? Learn in this blog post how to calculate the speed of a duty cycle with an average of 1000 loops!
Latest Posts
Requirements
PYTHON3-LIBRPIPLC
In order to do a speed test of the Raspberry PLC outputs, we will need to download the python3-librpiplc. You can check the Python library for Raspberry PLC blog post to help you download the library.
Testing
1. Once the library is already installed, let's create a new file called test.py:
nano test.py
2. Then, copy this code:
from rpiplc_lib import rpiplc
import time
def main():
    rpiplc.init("RPIPLC_21")
    rpiplc.pin_mode("Q0.0", 1)
    rpiplc.pin_mode("Q0.1", 1)
    rpiplc.pin_mode("Q0.2", 1)
    rpiplc.pin_mode("Q0.3", 1)
    rpiplc.pin_mode("Q0.4", 1)
    rpiplc.pin_mode("A0.5", 1)
    rpiplc.pin_mode("A0.6", 1)
    rpiplc.pin_mode("A0.7", 1)
    start = time.time()
    for i in range(1000) :
        rpiplc.digital_write("Q0.0", rpiplc.HIGH)
        rpiplc.digital_write("Q0.0", rpiplc.LOW)
        rpiplc.digital_write("Q0.1", rpiplc.HIGH)
        rpiplc.digital_write("Q0.1", rpiplc.LOW)
        rpiplc.digital_write("Q0.2", rpiplc.HIGH)
        rpiplc.digital_write("Q0.2", rpiplc.LOW)
        rpiplc.digital_write("Q0.3", rpiplc.HIGH)
        rpiplc.digital_write("Q0.3", rpiplc.LOW)
        rpiplc.digital_write("Q0.4", rpiplc.HIGH)
        rpiplc.digital_write("Q0.4", rpiplc.LOW)
        rpiplc.digital_write("A0.5", 4095)
        rpiplc.digital_write("A0.5", 0)
        rpiplc.digital_write("A0.6", 4095)
        rpiplc.digital_write("A0.6", 0)
        rpiplc.digital_write("A0.7", 4095)
        rpiplc.digital_write("A0.7", 0)
    end = time.time()
    res = ( int(end) - int(start) ) / 1000
    print(res)
if __name__ == "__main__":
    main()
Explanation
- First of all, we are going to import the rpiplc library.
- Then, we are going to define the pin mode of the outputs to 1, in order to set them as outputs and not inputs.
- After that, we will loop 1000 times for 1000 duty cycles.
- In order to measure this time, we will get the time before doing the loop, and the same once it is done. Then, we will subtract the end and the start time, and we will divide it by 1000, to get the speed of the duty cycle.
- Finally, we will print the result, and we will execute the main function to run everything explained.
Run the test
Now, run the following command in order to get the speed resolution for the outputs when all of them are running:
python3 test.py

Digital outputs
If you would like to know the speed of the outputs when only all of the digital outputs are running, just comment on the analog outputs, and run again the application.
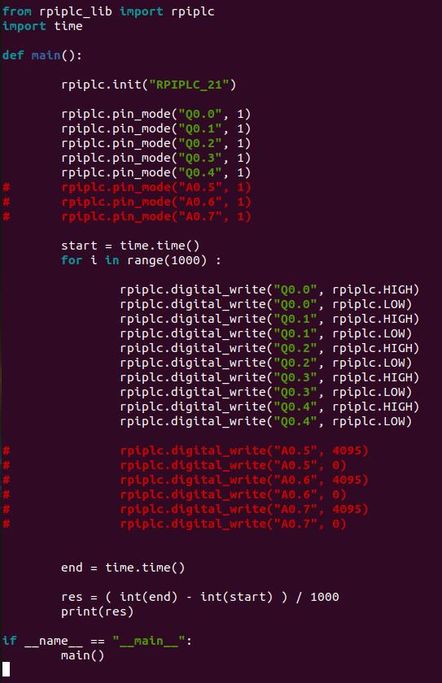
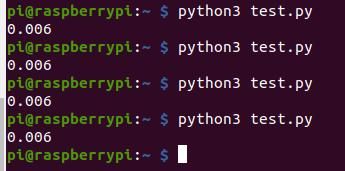
Analog outputs
And the opposite with the analog outputs: If you would like to know the speed of the outputs when only all of the analog outputs are running, just comment on the digital outputs, and run again the application.
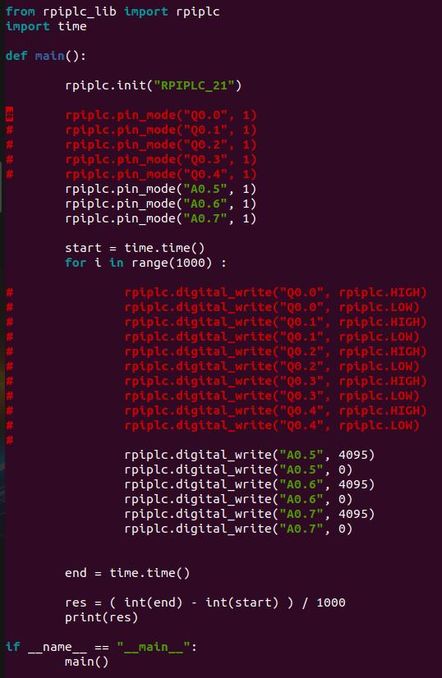
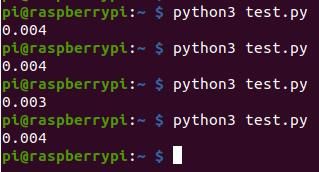
Speed Test Raspberry Pi PLC Outputs