Introduction
Being able to store data on the ESP32 without the need for an SD card is very useful in order not to lose track of states and variables when there is a power failure or an unwanted reset of the microcontroller.
The ESP32 microcontroller has a specific memory space designed for this purpose. Non-volatile storage (NVS) retains data through reboots and system power loss events (using a portion of the NVS).
With this method, more than 2 KBytes of data can be stored. It is important to note that NVS flash memory is primarily intended for storing small amounts of configuration data, such as WiFi credentials or application-specific settings.
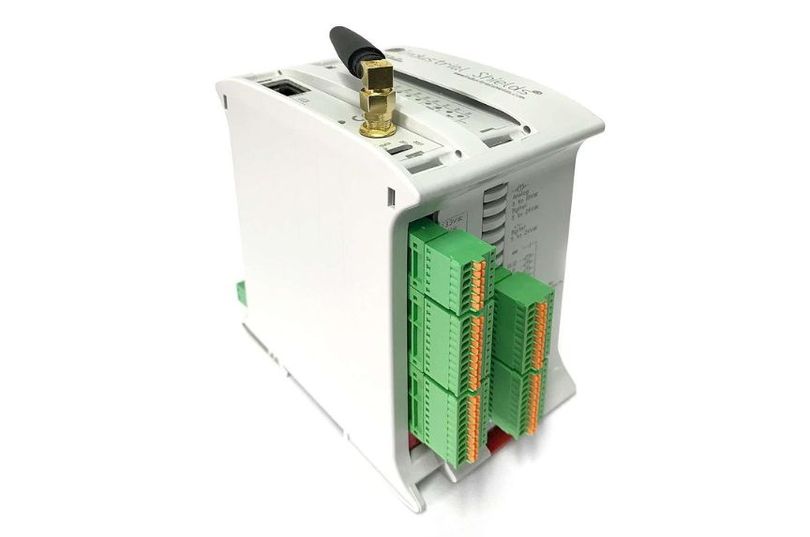
Requirements
Programmation
The code that will be shown is:
#include <Preferences.h>
Preferences p;
Preferences q;
void setup() {
Serial.begin(115200);
p.begin("test-1", false);
q.begin("test-2", false);
unsigned int counter1 = p.getUInt("counter", 0);
unsigned int counter2 = q.getUInt("counter", 0);
counter1 = counter1 + 1;
counter2 = counter2 + 2;
Serial.printf("Current counter1 value: %u\n", counter1);
Serial.printf("Current counter2 value: %u\n", counter2);
p.putUInt("counter", counter1);
q.putUInt("counter", counter2);
p.end();
q.end();
Serial.println("Restarting");
ESP.restart();
}
void loop() {}
Breakdown
Let's break down the code:
#include <Preferences.h>
Preferences p;
Preferences q;
First, we include the Preferences library that will be used to read and write to the NVS and objects p and q are defined.
p.begin("test-1", false);
q.begin("test-2", false);
Here we open p and q with the namespaces "test-1" and "test-2" respectively with Read/ Write permissions. If we only want Read, then the second parameter should be true:
p.begin("test-1", true);
Each namespace has its own key values, so repeating the key "counter" in both namespaces will not change anything.
unsigned int counter1 = p.getUInt("counter", 0);
unsigned int counter2 = q.getUInt("counter", 0);
The getUInt(key_name, default_value) function returns the value of the specified key. If the key does not exist, it returns the default_value (in this case, 0).
counter1 = counter1 + 1;
counter2 = counter2 + 2;
Serial.printf("Current counter1 value: %u\n", counter1);
Serial.printf("Current counter2 value: %u\n", counter2);
Here, variables counter1 (from namespace "test-1") and counter2 (from namespace "test-2") are incremented and then printed in the serial port.
p.putUInt("counter", counter1);
q.putUInt("counter", counter2);
Then we save the variables to their respective key and namespace using putUInt function.
p.end();
q.end();
Serial.println("Restarting");
ESP.restart();
Finally, we close both namespaces and we perform a reset on the ESP32 board.
After a few resets, you will see something like this:
As expected, counter1 increments by 1, while counter2 increments by 2 each time it is reset.
Additional functions
The following function can be used to delete the desired namespace:
p.clear();
If you only want to delete a key-value pair, you will use:
p.remove(key);
Find the Preferences library API here >>
To completely erase the NVS flash memory, execute the following code:
#include <nvs_flash.h>void setup() {nvs_flash_erase(); // Erase the NVS partitionnvs_flash_init(); // Initialize the NVS partitionwhile(true);}void loop() {}
Conclusion
The ESP32 microcontroller’s NVS is a useful way to store data without an SD card. It can keep important data even when power is lost or reset. It can store more than 2 KBytes of data, which is enough for configuration data like WiFi settings.
The Preferences library and its functions let users access and modify data in different parts of the NVS. Users can also delete or erase data as needed. Using the NVS on the ESP32 improves reliability and data persistence, making applications more stable and consistent even in difficult situations.
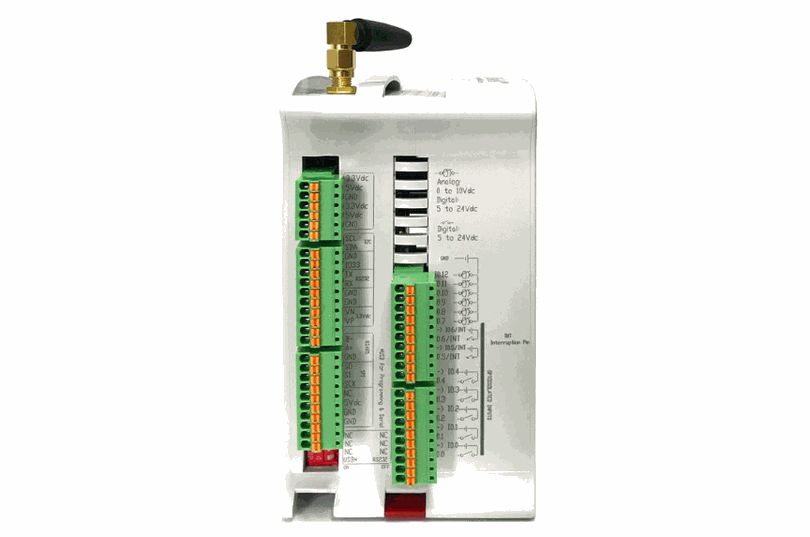
How to use ESP32 NVS memory to store data permanently