Introduction
In this post, we are going to explain how to do the basics in order to work with the analog inputs of Industrial Shields Raspberry Pi programmable logic controllers. By reading this post, you will be able to understand how to connect, configure and work with the inputs of your industrial Raspberry Pi PLC controller.
Previous readings
We recommend you read the following posts to understand the programming of this blog. We have used the following blog posts for this example:
How to access the Raspberry Pi based PLCÂ Â Â Read >>
How to change the IP in Windows and Linux   Read >>
Requirements
To work with analog outputs, you will need any of our industrial controllers for industrial automation:
Input types
On all Industrial Shields PLCs, analog inputs can work at:
0 Vdc - 10 Vdc input
Each of them has a particular drawing in the case of the PLC:
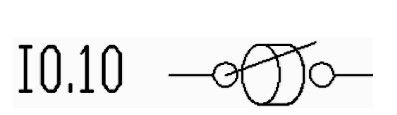
0 - 10 Vdc Analog Input
Software
How to work with Bash Scripts
Analog/Digital Shields
> cd /home/pi/test/analog
Relay Shield
> cd /home/pi/test/relay
The get-digital-input script will show the value of the selected input pin. Only the pin we are going to work with will be provided. The return value will be in the range of 0 (0Vdc) to 2047 (10 Vdc). In order to call the function, we will do the following:
> ./get-analog-input <input>
Example for the I0.12 input returning a True value if it's receiving 10Vdc:
> ./get-analog-input I0.12
2047
How to work with Python
The bash commands are the base for working easily with the industrial Raspberry PLC. In order to work with python files, if you want to interact with the IOs of the PLC, you will have to call these scripts.
To edit the files you will work with the Nano editor included by default and Python3.
nano analog_inputs.py
Python allows you to execute a shell command that is stored in a string using the subprocess library. In order to work with it, you will have to import it at the start of the file.
import subprocess
In this example, you will be going to read the input given of the pin I0.12 of the Raspberry Pi PLC. In order to do it, you will implement a loop that will be constantly reading the input value.
import subprocess import time def str2dec(string): return (string[0:-1]) def adc(value): return (10*int(str2dec(value)))/4096 if __name__ == "__main__": print("Start") while True: try: x = subprocess.run(["./get-analog-input","I0.12"], stdout=subprocess.PIPE, text=True): print(adc(x.stdout)) time.sleep(1) except KeyboardInterrupt: print("\nExit") break
 In order to execute the Python program, you will call it as follow:
> python3 analog_inputs.py
To exit the program, just press ^C.
Basics about analog inputs of Raspberry PLC